Create a DJ Mixer with Web Audio API
Date: January 03, 2023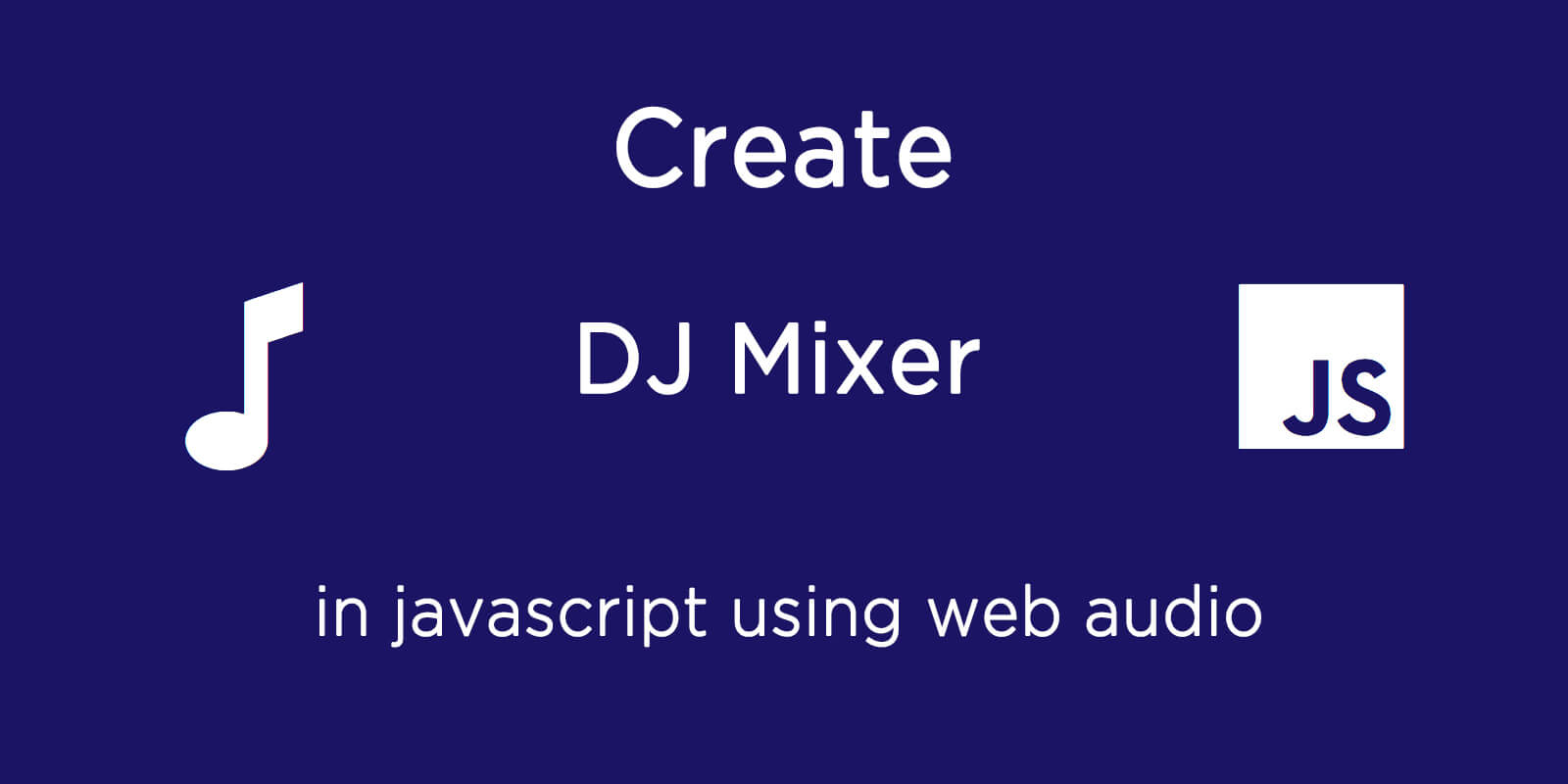
How to create a simple DJ Mixer using Web Audio API.
Below you will find sample code on how to use the Web Audio API to create a DJ Mixer that has a crossfade feature between two tracks as well as a couple audio effects.
JavaScript
// Create an audio context
var audioCtx = new (window.AudioContext || window.webkitAudioContext)();
// Create two audio sources
var track1 = new Audio('track1.mp3');
var track2 = new Audio('track2.mp3');
// Create gain nodes for each track
var gainNode1 = audioCtx.createGain();
var gainNode2 = audioCtx.createGain();
// Connect the audio sources to the gain nodes
var source1 = audioCtx.createMediaElementSource(track1);
source1.connect(gainNode1);
var source2 = audioCtx.createMediaElementSource(track2);
source2.connect(gainNode2);
// Set the initial gain (volume) for each track
gainNode1.gain.value = 0;
gainNode2.gain.value = 0;
// Create effects nodes
var biquadFilter1 = audioCtx.createBiquadFilter();
biquadFilter1.type = 'lowpass';
biquadFilter1.frequency.value = 1000;
var biquadFilter2 = audioCtx.createBiquadFilter();
biquadFilter2.type = 'highpass';
biquadFilter2.frequency.value = 1000;
// Connect the gain nodes to the effects
gainNode1.connect(biquadFilter1);
gainNode2.connect(biquadFilter2);
// Connect the effects to the destination (speakers or headphones)
biquadFilter1.connect(audioCtx.destination);
biquadFilter2.connect(audioCtx.destination);
// Create a function to apply effects to a track
function applyEffect(track, effect) {
if (track === 1) {
biquadFilter1.type = effect;
} else if (track === 2) {
biquadFilter2.type = effect;
}
}
// Create a function to fade in a track
function fadeInTrack(track, fadeTime) {
var currentTime = audioCtx.currentTime;
if (track === 1) {
gainNode1.gain.setValueAtTime(0, currentTime);
gainNode1.gain.linearRampToValueAtTime(1, currentTime + fadeTime);
} else if (track === 2) {
gainNode2.gain.setValueAtTime(0, currentTime);
gainNode2.gain.linearRampToValueAtTime(1, currentTime + fadeTime);
}
}
// Example usage: apply a lowpass effect to track 1, fade in track 1 over 5 seconds
applyEffect(1, 'lowpass');
fadeInTrack(1, 5);
Links: Create a DJ Mixer with Web Audio API