Draw waveform from micrphone to canvas
Date: January 10, 2023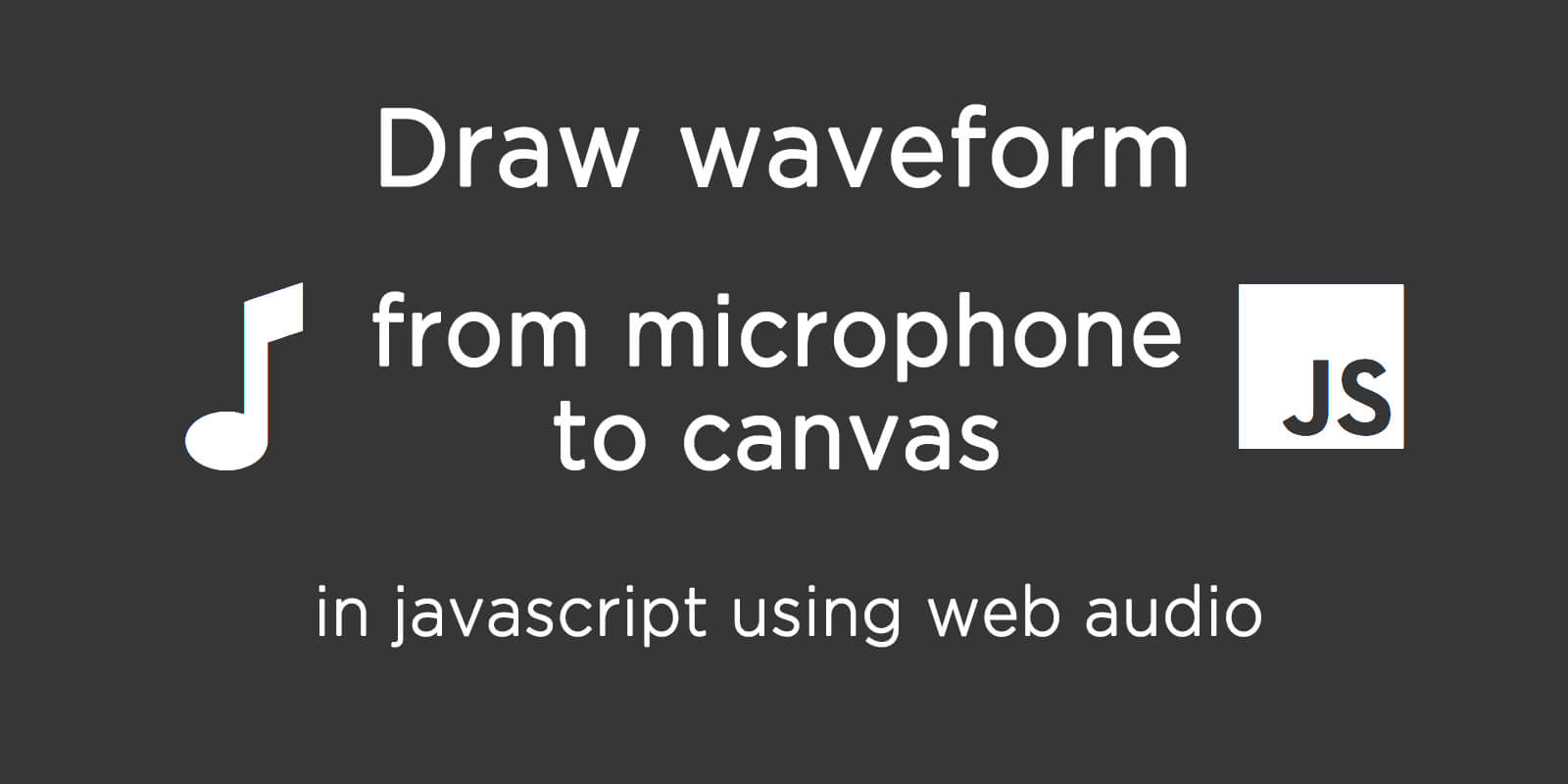
How to draw waveform from microphone audio to canvas.
Below you will find sample code on how to use the Web Audio API to capture audio from a microphone and draw the audio waveform using canvas.
JavaScript
navigator.mediaDevices.getUserMedia({ audio: true }).then(function(stream) {
var context = new AudioContext();
var source = context.createMediaStreamSource(stream);
var analyser = context.createAnalyser();
source.connect(analyser);
var bufferLength = analyser.frequencyBinCount;
var dataArray = new Float32Array(bufferLength);
var canvas = document.getElementById("waveform");
var canvasCtx = canvas.getContext("2d");
function draw() {
requestAnimationFrame(draw);
analyser.getFloatTimeDomainData(dataArray);
canvasCtx.fillStyle = "rgb(200, 200, 200)";
canvasCtx.fillRect(0, 0, canvas.width, canvas.height);
canvasCtx.lineWidth = 2;
canvasCtx.strokeStyle = "rgb(0, 0, 0)";
canvasCtx.beginPath();
var sliceWidth = canvas.width * 1.0 / bufferLength;
var x = 0;
for (var i = 0; i < bufferLength; i++) {
var v = dataArray[i] * 200.0;
var y = (v / 2) + 100;
if (i === 0) {
canvasCtx.moveTo(x, y);
} else {
canvasCtx.lineTo(x, y);
}
x += sliceWidth;
}
canvasCtx.lineTo(canvas.width, canvas.height / 2);
canvasCtx.stroke();
};
draw();
});
Links: Draw waveform from micrphone to canvas