Fetch API
Date: December 21, 2018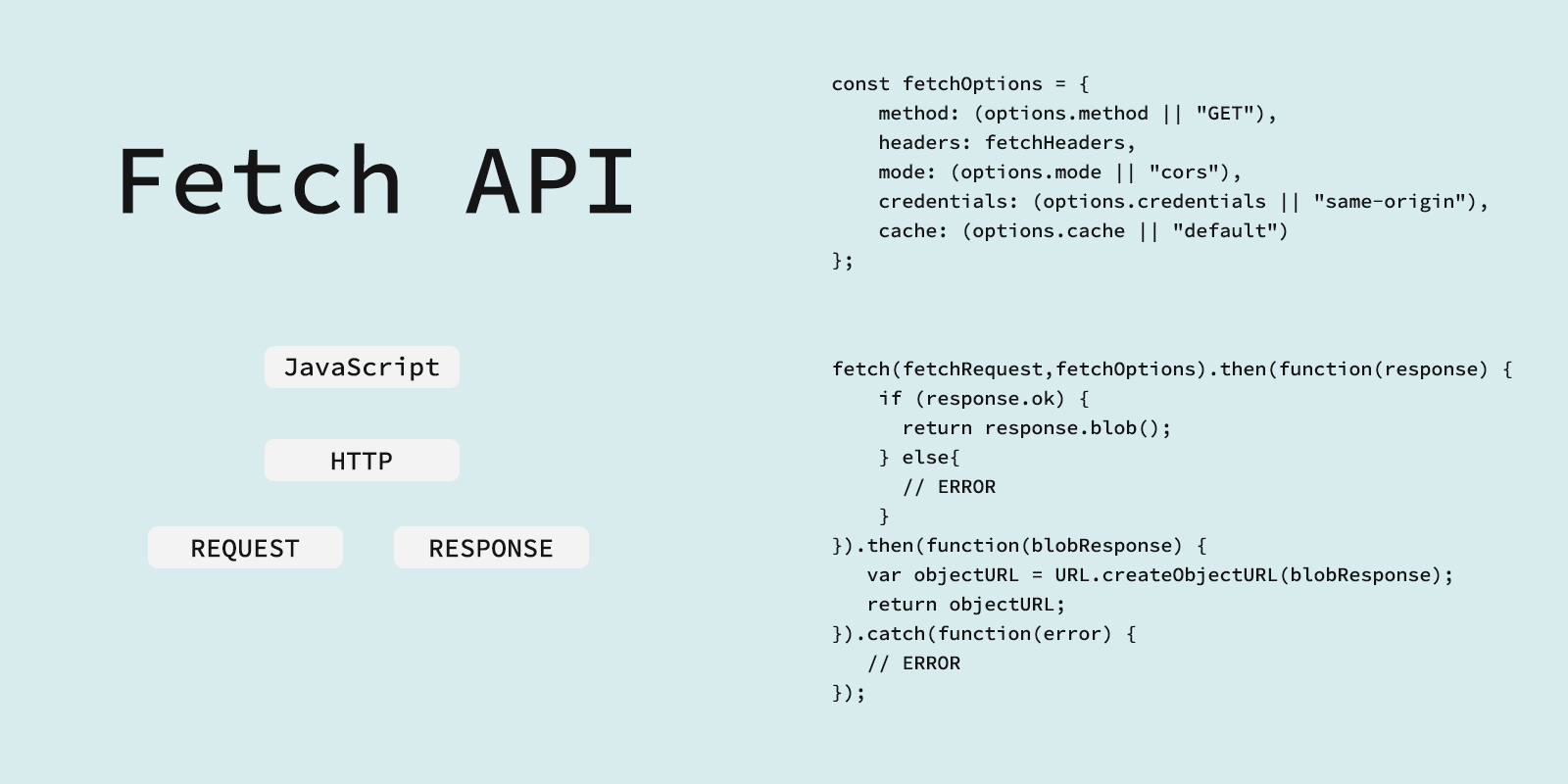
The Fetch API is a new JavaScript interface that allows editing parts of the HTTP requests and responses. It also supports fetching resources asynchronously by also allowing for custom credentials and headers to be specified. This functionality can also be achieved with XMLHttpRequest, however the Fetch API provides an easier way to be used with Service Workers.
Below you will find sample code on how to use the Fetch API with sample json data from waveform.al.
JavaScript
dofetch({
url: "https://www.waveform.al/sample.json",
requestHeaders: [{
header: {
"Content-Type":"application/json"
}
}]
});
function dofetch(options) {
if (self.fetch) {
const instance = function(message){
console.log(message);
};
instance.fireEvent = function(type,message,output){
console.log(message);
console.log(type);
console.log(output);
};
const fetchRequest = new Request(options.url);
let fetchHeaders = new Headers();
// check if headers
if (options) {
if (options.requestHeaders) {
// add custom request headers
options.requestHeaders.forEach(header => {
fetchHeaders.append(header.key, header.value);
});
}
}
// set default fetch options
const fetchOptions = {
method: (options.method || "GET"),
headers: fetchHeaders,
mode: (options.mode || "cors"),
credentials: (options.credentials || "same-origin"),
cache: (options.cache || "default")
};
// do the fetch
fetch(fetchRequest,fetchOptions).then(function(response) {
if (response.ok) {
return response.blob();
} else{
instance.fireEvent('HTTP error status ', response.status)
}
}).then(function(blobResponse) {
var objectURL = URL.createObjectURL(blobResponse);
instance.fireEvent('load', objectURL);
instance.fireEvent('success', fetchRequest.response, objectURL);
}).catch(function(error) {
instance.fireEvent('error', error.message)
});
// return the fetch
instance.fetchRequest = fetchRequest;
return instance;
} else{
instance.fireEvent('error', 'fetch api not supported');
}
}
Links: Fetch API