Play Do Re Mi Fa So in JavaScript
Date: January 20, 2023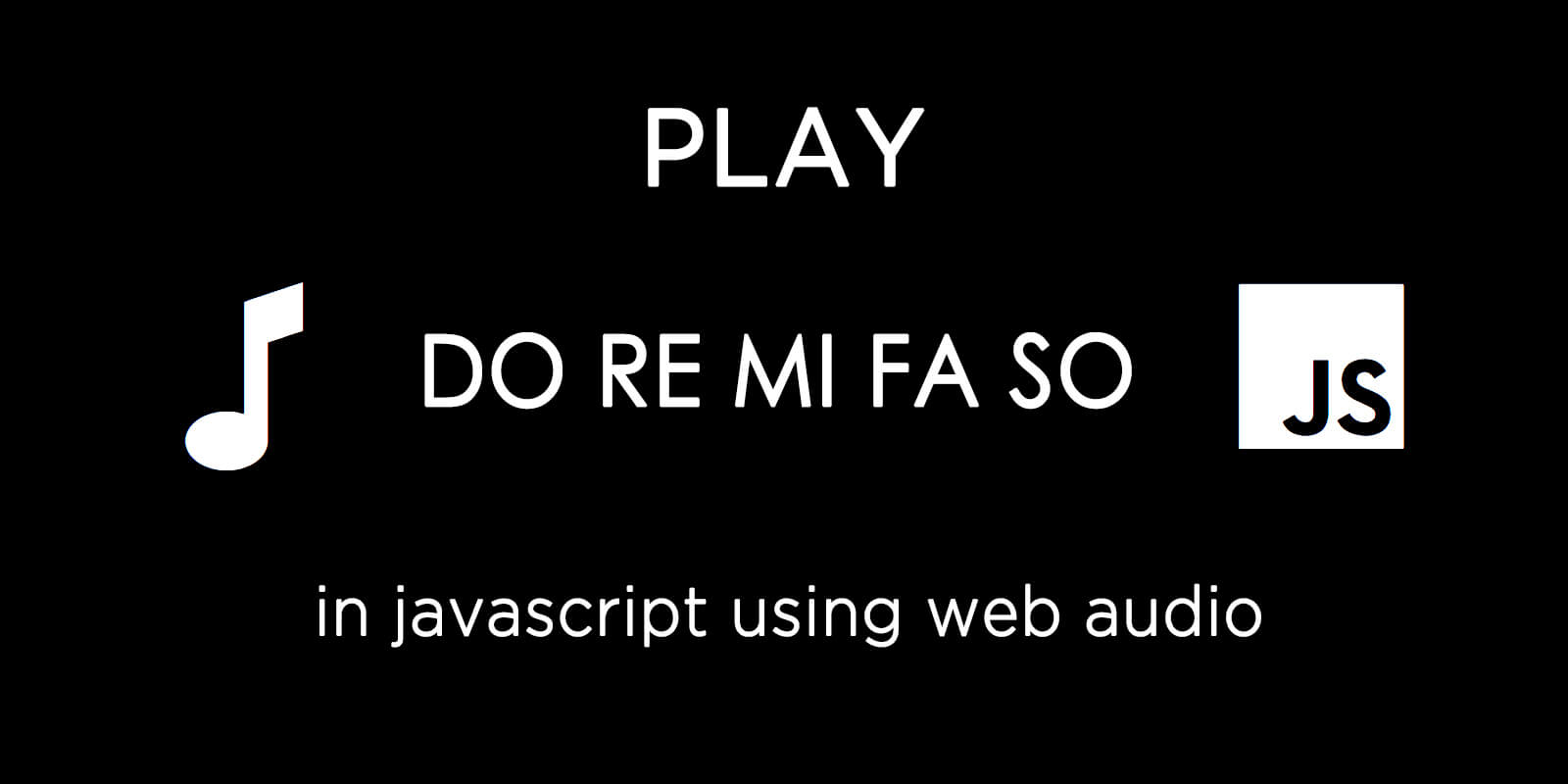
How to play Do Re Mi Fa So melody using Web Audio API.
Below you will find sample code on how to use the Web Audio API to play Do Re Mi Fa So melody in JavaScript.
JavaScript
var context = new (window.AudioContext || window.webkitAudioContext)();
var doReMiFaSo = [
{note: "C4", start: 0, duration: 0.5},
{note: "D4", start: 0.5, duration: 0.5},
{note: "E4", start: 1, duration: 0.5},
{note: "F4", start: 1.5, duration: 0.5},
{note: "G4", start: 2, duration: 0.5},
{note: "A4", start: 2.5, duration: 0.5},
{note: "B4", start: 3, duration: 0.5},
{note: "C5", start: 3.5, duration: 0.5}
];
var tempo = 100; // beats per minute
var quarterNoteTime = 60 / tempo;
doReMiFaSo.forEach(function(note) {
var startTime = note.start * quarterNoteTime;
var endTime = startTime + note.duration * quarterNoteTime;
var oscillator = context.createOscillator();
oscillator.type = "sine";
var noteFrequency = getFrequency(note.note);
oscillator.frequency.value = noteFrequency;
oscillator.connect(context.destination);
oscillator.start(context.currentTime + startTime);
oscillator.stop(context.currentTime + endTime);
});
function getFrequency(note) {
switch (note) {
case "C4":
return 261.63;
case "D4":
return 293.66;
case "E4":
return 329.63;
case "F4":
return 349.23;
case "G4":
return 392.00;
case "A4":
return 440.00;
case "B4":
return 493.88;
case "C5":
return 523.25;
default:
return 0;
}
}
Links: Play Do Re Mi Fa So in JavaScript