Record Microphone with Web Audio API
Date: January 07, 2023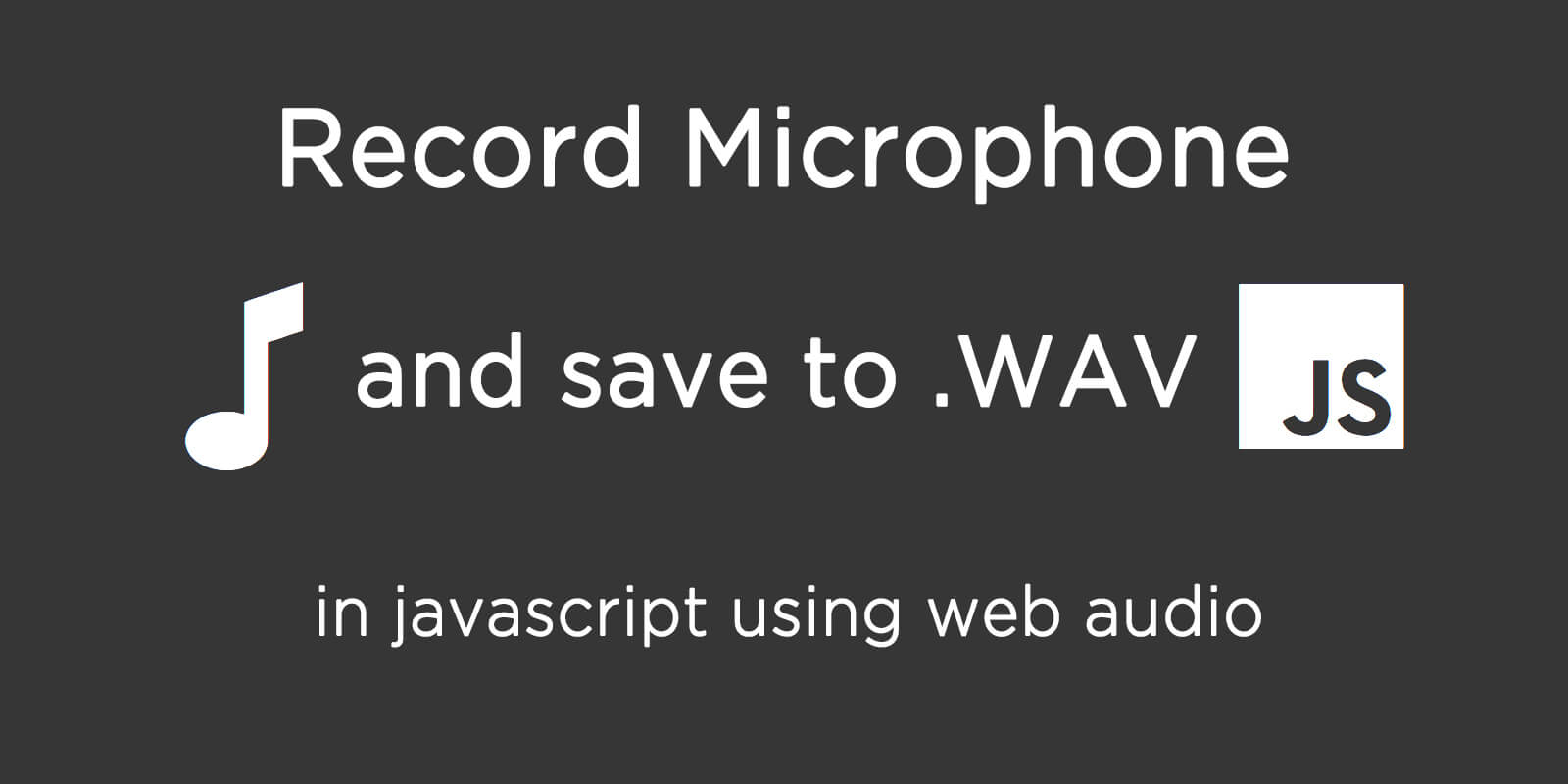
How to record microphone and save as wav format using Web Audio API.
Below you will find sample code on how to record audio from microphone and save the audio data as a wav file format.
processor.js
JavaScript
class YourWorkletProcessor extends AudioWorkletProcessor {
process(inputs, outputs, parameters) {
// Get the audio data from the input buffer
const inputData = inputs[0][0];
// Output the processed data
outputs[0].set(inputData);
// Return true to indicate that the node should continue processing
return true;
}
}
registerProcessor('your-worklet-processor-name', YourWorkletProcessor);
recorder.js
JavaScript
let recordingBuffer = [];
// Request microphone access
audioWorklet.addModule('processor.js')
.then(() => {
navigator.mediaDevices.getUserMedia({ audio: true })
.then(stream => {
// Create an AudioContext
const audioContext = new AudioContext();
// Create a MediaStreamSource node to feed the stream into the audio context
const source = audioContext.createMediaStreamSource(stream);
// Create a AudioWorkletNode to process the audio data
const workletNode = new AudioWorkletNode(audioContext, 'your-worklet-processor-name');
// Connect the source to the script processor
source.connect(workletNode);
// Connect the script processor to the destination (the speakers)
workletNode.connect(audioContext.destination);
// Function to export the recording as a WAV file
function exportWAV() {
// Create a new AudioContext to process the data
const exportAudioContext = new AudioContext();
// Create a Float32Array to hold the entire recording
const recordedData = mergeBuffers(recordingBuffer, recordingBuffer.length);
// Create a buffer source to process the data
const bufferSource = exportAudioContext.createBufferSource();
const buffer = exportAudioContext.createBuffer(1, recordedData.length, exportAudioContext.sampleRate);
buffer.getChannelData(0).set(recordedData);
bufferSource.buffer = buffer;
// Use the built-in encodeWAV method to create the WAV file
const encodedWAV = exportAudioContext.encodeWAV(bufferSource.buffer);
// Create a Blob from the encoded WAV data
const blob = new Blob([encodedWAV], { type: 'audio/wav' });
// Create a link to download the file
const downloadLink = document.createElement('a');
downloadLink.href = URL.createObjectURL(blob);
downloadLink.download = 'recording.wav';
// Add the link to the DOM and simulate a click to start the download
document.body.appendChild(downloadLink);
downloadLink.click();
// Remove the link from the DOM
document.body.removeChild(downloadLink);
}
})
.catch(err => {
console.error('Failed to get microphone access:', err);
});
});
Links: Record Microphone with Web Audio API